The Power of React.js: A Complete Guide forDevelopers
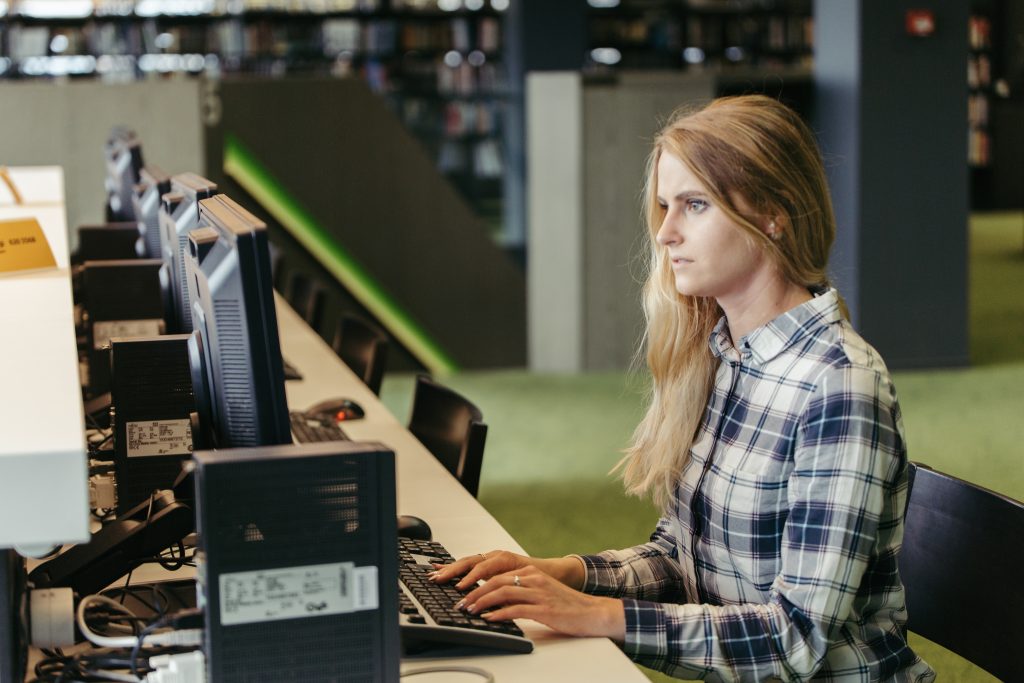
The Power of React.js: A Complete Guide for Developers
Introduction
In the ever-evolving world of web development, React.js has emerged as one of the most powerful and widely used JavaScript libraries. Whether you’re building a small business website, a complex enterprise application, or an interactive user interface, React.js offers a flexible, efficient, and scalable solution. In this article, we’ll explore the key features, advantages, and best practices of React.js development, making it an essential read for both beginners and experienced developers.
What is React.js?
React.js is an open-source JavaScript library developed by Facebook (now Meta) for building user interfaces, particularly single-page applications (SPAs). It allows developers to create reusable UI components that update dynamically without reloading the page, enhancing performance and user experience. Since its release in 2013, React.js has become the go-to library for front-end development, powering applications like Facebook, Instagram, Airbnb, and Netflix.
Why Choose React.js for Web Development?
1. Component-Based Architecture
React follows a component-based architecture, where the UI is broken down into small, reusable pieces called components. These components make the code more manageable, scalable, and maintainable, allowing developers to build complex applications efficiently.
2. Virtual DOM for Faster Performance
React uses a Virtual DOM (Document Object Model) to enhance performance. Instead of directly manipulating the browser’s DOM, React first updates a virtual representation and then synchronizes only the necessary changes. This optimization significantly improves the speed and efficiency of rendering UI components.
3. Declarative Syntax
With React’s declarative syntax, developers can describe what the UI should look like at any given state. This makes debugging easier and ensures that the UI remains predictable, even as the application grows in complexity.
4. JSX – JavaScript XML
React uses JSX (JavaScript XML), a syntax extension that allows developers to write HTML directly within JavaScript. JSX makes code more readable and enhances the development process by integrating UI components seamlessly.
5. Strong Community Support & Ecosystem
React has a vast community of developers, making it easy to find solutions, libraries, and third-party integrations. Frameworks like Next.js and tools like Redux and React Router further extend its capabilities, making React development even more powerful.
Getting Started with React.js
To start building applications with React.js, follow these simple steps:
Step 1: Install Node.js
Before installing React, you need Node.js and npm (Node Package Manager). You can download them from nodejs.org.
Step 2: Create a React App
React provides a command-line tool called Create React App (CRA) for setting up a new project quickly:
npx create-react-app my-app
cd my-app
npm start
This sets up a fully configured React application with all dependencies.
Step 3: Understand the Folder Structure
Once installed, the project directory will have files like:
src/ – Contains the main React components and logic.
public/ – Holds static files such as images and HTML templates.
package.json – Manages project dependencies and scripts.
Step 4: Create Your First React Component
React components can be functional or class-based. Here’s an example of a simple functional component:
import React from 'react';
function Greeting() {
return <h1>Hello, Welcome to React.js!</h1>;
}
export default Greeting;
This component can be used in App.js like this:
import React from 'react';
import Greeting from './Greeting';
function App() {
return (
<div>
<Greeting />
</div>
);
}
export default App;
Best Practices for React Development
1. Keep Components Small and Reusable
Breaking UI elements into smaller, reusable components improves maintainability and readability. A well-structured React project consists of atomic components that serve specific purposes.
2. Use State Management Efficiently
React has a built-in useState hook for managing state in functional components. For large-scale applications, tools like Redux, Zustand, or Context API help manage state more effectively.
3. Optimize Performance with Memoization
React provides React.memo and useMemo to prevent unnecessary re-renders, improving performance:
import React, { useMemo } from 'react';
const ExpensiveCalculation = ({ value }) => {
const result = useMemo(() => computeHeavyTask(value), [value]);
return <p>{result}</p>;
};
4. Follow Proper Folder Structure
Organizing your React project with a clear folder structure enhances collaboration and scalability. A common pattern is:
src/
├── components/
├── pages/
├── services/
├── utils/
├── assets/
5. Implement Lazy Loading
React’s lazy loading improves application speed by loading components only when needed:
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
Future of React.js
React.js continues to evolve with new features like React Server Components, Concurrent Mode, and React 19 updates. Its ability to build progressive web apps (PWAs), mobile applications with React Native, and seamless integrations with backend frameworks makes it a dominant force in web development.
Conclusion
React.js is a powerful, flexible, and efficient library that simplifies front-end development. With features like component-based architecture, Virtual DOM, and JSX, it provides an excellent foundation for building modern web applications. Whether you’re a beginner or an experienced developer, mastering React.js opens doors to endless possibilities in web development.
If you’re looking to build high-performance, scalable web applications, React.js is the ultimate choice! Start your journey today and unlock the full potential of modern front-end development. 🚀